Displaying Future Weather Data
Now, tap Forecast Report to see the weather report for the coming days. What happened? It just keeps loading, right? That’s because you haven’t connected the screen to WeatherService
.
So, open weather_forecast_screen.dart inside the weather package, and add these import
statements:
import 'weather_service.dart';
import '../location/location_data.dart';
import '../location/location_picker.dart';
import '../location/inherited_location.dart';
Then, add the following methods below build()
of _WeatherForecastScreenState
:
@override
void didChangeDependencies() {
fetchWeatherForecast(InheritedLocation.of(context).location);
super.didChangeDependencies();
}
void fetchWeatherForecast(LocationData? loc) async {
if (loc == null) return;
forecasts = WeatherService.instance().getForecasts(loc);
}
In the AppBar
in the Scaffold
of the same _WeatherForecastScreenState
, pass an actions
property, like so:
appBar: AppBar(
...
actions: const [LocationPicker()],
),
Rerun the project. Tap Forecast Report again, and you’ll see this:
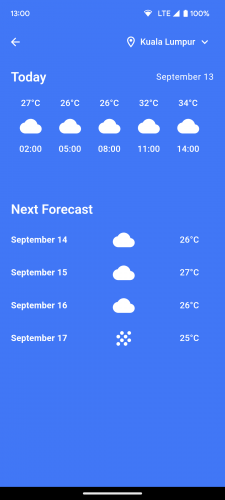
On the forecast screen, changing the location will update the weather data. If you return to the current weather screen, it’ll display the location you picked on the forecast screen and its weather data. That’s the power of InheritedWidget!
Where to Go From Here?
Congratulations on completing this tutorial!
The final directory contains the full code used in this tutorial, and you can find it in the zipped file you downloaded earlier. You can still download it by clicking the Download materials button at the top or bottom of this tutorial.
In this tutorial, you learned about InheritedWidgets
and how it differs from a stateless and a stateful widget. You also got your hands dirty by propagating the location state with InheritedLocation
and managing it with LocationProvider
. You can learn more about InheritedWidget
by watching InheritedWidgets | Decoding Flutter from the Flutter team.
We hope you enjoyed this tutorial. If you have any questions or comments, please join the forum discussion below!