Remote Image Loading
What if your application requires loading external images inside each individual cell? This task generally requires subclassing AsyncTask
and firing off an image download task for each cell as the user scrolls down the list and back up.
Having implemented a custom solution for this in the past, I can tell you that it gets complicated. For example, you’d need to cache images in memory as each download is successfully completed, while making sure you’re not firing off multiple download tasks for the same image file download.
Fortunately, there is a fantastic library available from Square called Picasso that solves many of the common issues with list views and image downloads. To install the library, open up build.grade (Module: app) in your project and add the following line of code inside dependencies
:
dependencies {
...
compile 'com.squareup.picasso:picasso:2.5.2'
}
Your build file should look like this:
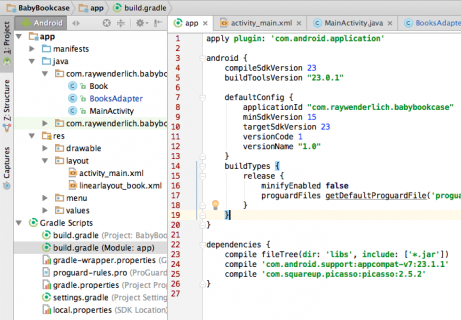
Next, click the Sync Now link to install Picasso and update your project:

After successfully syncing your project, you can let Picasso do all the heavy lifting of downloading your images. To do this, comment out the line in BooksAdapter.getView()
where the cover art is set and include Picasso like this:
// make sure to comment out this image setter
//viewHolder.imageViewCoverArt.setImageResource(book.getImageResource());
Picasso.with(mContext).load(book.getImageUrl()).into(viewHolder.imageViewCoverArt);
Build and run, then scroll through the GridView to test the external image loading. Note that the images are still the same for each book, but they are coming from a remote URL now.
Where to Go From Here
Congratulations! You’ve conquered the powerful GridView
component, created a library for your favorite kids’ books and learned how to do the following:
- Add a
GridView
to your layouts.
- Create an adapter to provide your
GridView
with the proper cell views.
- React to item selections.
- Persist selections for orientation changes.
- Customize the
GridView
.
- Optimize performance.
You can download the completed project here. If you’re curious about what else you can do with GridView
s, check out Google’s documentation.
I hope you’ve enjoyed this introduction to Android’s GridView
. If you have any questions, comments or feedback please join the forum discussion below.