Requesting App Ratings and Reviews Tutorial for iOS
In this tutorial, you’ll learn about requesting app ratings and reviews in your iOS applications using SKStoreReviewController and other available APIs. By Sanket Firodiya.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Requesting App Ratings and Reviews Tutorial for iOS
20 mins
Adding Review Request Logic in the Sample App
In the sample app, you’ll use a strategy where you will ask users for a rating once they have played at least three recordings. This number is deliberately low so that you can test the concept. In a real-world app, you would want to keep this number much higher for a similar user action.
Open AppStoreReviewManager.swift and replace requestReviewIfAppropriate()
with the following:
// 1.
static let minimumReviewWorthyActionCount = 3
static func requestReviewIfAppropriate() {
let defaults = UserDefaults.standard
let bundle = Bundle.main
// 2.
var actionCount = defaults.integer(forKey: .reviewWorthyActionCount)
// 3.
actionCount += 1
// 4.
defaults.set(actionCount, forKey: .reviewWorthyActionCount)
// 5.
guard actionCount >= minimumReviewWorthyActionCount else {
return
}
// 6.
let bundleVersionKey = kCFBundleVersionKey as String
let currentVersion = bundle.object(forInfoDictionaryKey: bundleVersionKey) as? String
let lastVersion = defaults.string(forKey: .lastReviewRequestAppVersion)
// 7.
guard lastVersion == nil || lastVersion != currentVersion else {
return
}
// 8.
SKStoreReviewController.requestReview()
// 9.
defaults.set(0, forKey: .reviewWorthyActionCount)
defaults.set(currentVersion, forKey: .lastReviewRequestAppVersion)
}
Breaking down the code above:
- Declare a constant value to specify the number of times that user must perform a review-worthy action.
- Read the current number of actions that the user has performed since the last requested review from the User Defaults.
- Increment the action count value read from User Defaults.
- Set the incremented count back into the user defaults for the next time that you trigger the function.
- Check if the action count has now exceeded the minimum threshold to trigger a review. If it hasn’t, the function will now return.
- Read the current bundle version and the last bundle version used during the last prompt (if any).
- Check if this is the first request for this version of the app before continuing.
- Ask StoreKit to request a review.
- Reset the action count and store the current version in User Defaults so that you don’t request again on this version of the app.
UserDefaults
to eliminate the need for using “stringly” typed keys when accessing values. This is a good practice to follow in order to avoid accidentally mistyping a key as it can cause hard-to-find bugs in your app. You can find this extension in UserDefaults+Key.swift.
UserDefaults
to eliminate the need for using “stringly” typed keys when accessing values. This is a good practice to follow in order to avoid accidentally mistyping a key as it can cause hard-to-find bugs in your app. You can find this extension in UserDefaults+Key.swift.
Putting It All Together
Build and run the app. This time, if you play a sound, it won’t immediately show the review prompt. Play the sound two more times and, only then, the app will present you with the rating prompt. You’ll also notice that the prompt won’t show again no matter how many recordings you play.
Click on the Chirper project icon in the Project navigator, then Chirper again under the list of presented targets. Update the value in the Build field to update the value of CFBundleVersion
in your apps Info.plist.
Build and run the app. Again, play more recordings. Once the .reviewWorthyActionCount
value exceeds three again, the version check in step 7 will succeed and, because you’ve changed the bundle version, you’ll be prompted again to give another rating for the new version of your app.
Manually Requesting a Review
So now you know how to prompt your users to provide a rating while using your app, you’ll move onto exploring how to let your users manually review the app if they wish to without having to wait for AppStoreReviewManager
to decide if they should or not.
First of all, you need to know the url for your app on the App Store. If your app is already live, then this is pretty easy; you can search for your app in Google and copy the url. Alternatively, you can find it on the App Store and tap the … button on the product page to reveal a Share App… option that allows you to copy the link to your clipboard.
If you’re still building the first version of your app and haven’t yet released it on the App Store, don’t worry; as long as you have already set up your app using App Store Connect, then you’ll still be able to get the link before you even submit your final to App Store Review. To find this link:
Writing a Review
Sharing Your App
Where to Go From Here?
.
.
The URL you end up obtaining will look something like this:
Before you continue, you’ll clean up the URL a little bit as some components are not required and can change over time. You can exclude the country code, app name and query parameter from the URL so that the above example would become the following:
The exact URL above is already included in the sample project, in SettingsViewController.swift. If you want to substitute the URL for your own app, go ahead and change the productURL
constant, otherwise leave it as it is and you’ll be reviewing the RWDevCon app. Be kind!
Now that you have a product URL for your app, you can use this to take your users directly to the Write a Review action in the App Store. All you have to do is append a query parameter with the name action
and the value write-review
to the product page URL; then, open it on an iOS device to go directly into the App Store app.
Add this functionality to the sample app!
Open SettingsViewController.swift and add the following code to writeReview()
:
The code above runs when the user taps the Write a Review cell in the Settings screen of the sample app. Going over the code:
Build and run the app onto your device. Tap the Settings icon in the nav bar and then Write a Review. You’ll be taken from the sample app and into the App Store where you’ll see the following screen:
When users are really enjoying your app, they might want to share the great experience they are having with their friends and family. Because there isn’t currently an easily discoverable way to share a link of your app directly, and you already have a link to your app’s product page, you might as well provide your users with a convenient way to share the link. After all, the more users you have, the merrier you will be!
You’re going to use a dedicated API for this called UIActivityViewController
. To do so, open SettingsViewController.swift and add the following code to share()
:
UIActivityViewController
works by accepting an array of “activity items”. It will then present a system interface with options to launch other apps installed on the device that have registered their own support for any of the provided activity item types.
In the code above, you provide the URL
object that represents the App Store product page and then modally present the activity view controller. Any apps or actions that can accept text or links will then be presented to you so that you can share the link however you like! You can even AirDrop it to another device to immediately open the App Store if you’d like.
Build and run your project onto either a physical device or simulator (the simulator will present fewer options) and you should see something like the following:
These are additional ways to improve the rating of your app and get happy users to spread the word about it!
You can download the completed version of the project using the Download Materials button at the top or bottom of this tutorial.
You have learned all about requesting app ratings and reviews for your app and how to do it at the right time. To take this even further, add SKStoreReviewController
to your apps in the next update that you release. Hopefully, you will see an uptick in the number of reviews and ratings and more traffic to your app on the App Store.
To learn more on this topic, these are few other good resources:
- Log in to App Store Connect
- Select My Apps
- Click on the app in the list App Information, where you will then find a link to View on App Store at the bottom of the page.
- Create an instance of
URLComponents
with the product page URL that you found earlier. URLComponents is a struct that assists with parsing and constructingURL
objects in a safe manner. It’s often good practice to useURLComponents
instead of just appending strings together. - Set the array of query items to contain a single item with the name and value to match the requirements that will trigger the App Store to open the prompt.
- Create a
URL
object based on the components that you modified in the last step. - Ask the shared
UIApplication
instance to open the given URL.
https://itunes.apple.com/us/app/rwdevcon-conference/id958625272?mt=8
https://itunes.apple.com/app/id958625272
// 1.
var components = URLComponents(url: productURL, resolvingAgainstBaseURL: false)
// 2.
components?.queryItems = [
URLQueryItem(name: "action", value: "write-review")
]
// 3.
guard let writeReviewURL = components?.url else {
return
}
// 4.
UIApplication.shared.open(writeReviewURL)
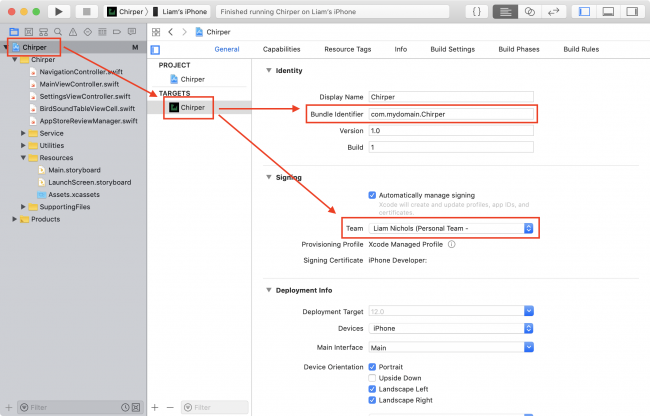
// 1.
let activityViewController = UIActivityViewController(
activityItems: [productURL],
applicationActivities: nil)
// 2.
present(activityViewController, animated: true, completion: nil)
- Create an instance of
URLComponents
with the product page URL that you found earlier. URLComponents is a struct that assists with parsing and constructingURL
objects in a safe manner. It’s often good practice to useURLComponents
instead of just appending strings together. - Set the array of query items to contain a single item with the name and value to match the requirements that will trigger the App Store to open the prompt.
- Create a
URL
object based on the components that you modified in the last step. - Ask the shared
UIApplication
instance to open the given URL.
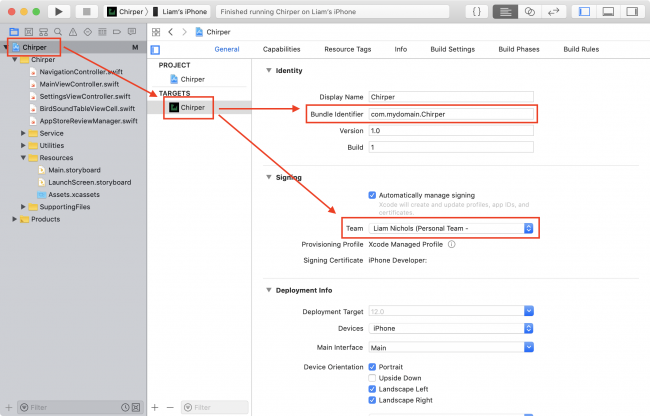
https://itunes.apple.com/us/app/rwdevcon-conference/id958625272?mt=8
https://itunes.apple.com/app/id958625272
// 1.
var components = URLComponents(url: productURL, resolvingAgainstBaseURL: false)
// 2.
components?.queryItems = [
URLQueryItem(name: "action", value: "write-review")
]
// 3.
guard let writeReviewURL = components?.url else {
return
}
// 4.
UIApplication.shared.open(writeReviewURL)
// 1.
let activityViewController = UIActivityViewController(
activityItems: [productURL],
applicationActivities: nil)
// 2.
present(activityViewController, animated: true, completion: nil)
- Requesting App Store Reviews
- Best Practices and What’s New with In-App Purchases
- Ray Wenderlich screencast on SKStoreReviewController
- App Store: Ratings, Reviews, and Responses
I hope you’ve enjoyed this tutorial; if you have any questions feel free to leave them in the discussion below! :]