Subtype Instances
Replace the code in convert.setOnClickListener
, and create two AcceptedCurrency
instances using the new function
convert.setOnClickListener {
val low = currencyFromSelection()
val high = currencyFromSelection()
}
These two values are instances of the same AcceptedCurrency
subtype, the one that’s selected in the spinner. You see here that unlike enum cases, you can create multiple instances of a sealed class subtype.
Finish off the convert button click listener by converting the amounts in the fields to amounts on the AcceptedCurrency
instances, and then show those amounts in dollars in the text views using the totalValueInDollars()
function of the parent sealed class:
low.amount = lowAmount.text.toString().toDouble()
high.amount = highAmount.text.toString().toDouble()
lowAmountInDollars.text = String.format("$%.2f", low.totalValueInDollars())
highAmountInDollars.text = String.format("$%.2f", high.totalValueInDollars())
Build and run the app.
Enter low and high values and choose a non-dollar currency. Then click convert and you’ll see the equivalent dollar amounts.
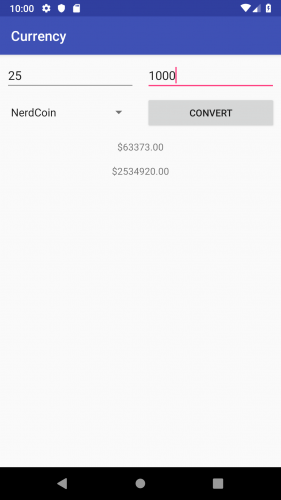
Congratulations! You’re now the possessor of some supreme knowledge on Kotlin sealed classes!
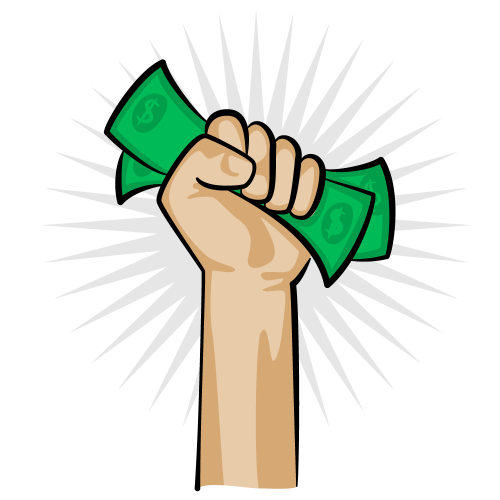
Where to Go From Here?
You can use the Download Materials button at the top or bottom of the tutorial to access the final version of the currency converter app.
You’ve seen how sealed classes let you build restricted hierarchies for a finite set of states, in this case a set of currencies.
Another common use for sealed classes is tracking the loading state of a screen, e.g. loading, empty, or done.
Sealed classes come in very handy in architecture patterns like Model-View-Intent, in which a stream of user intents (be careful—not the Android Intent
class) get translated to different types of representations as they are processed by the app. Sealed classes are a match for handling those different representations.
Check out the official documentation to learn more about sealed classes.
If you have any questions or comments, please join the discussion below!