Block Unwanted Swaps
Time to put this list of possible moves to good use. Add the following method to Level.swift:
func isPossibleSwap(_ swap: Swap) -> Bool {
return possibleSwaps.contains(swap)
}
This looks to see if the set of possible swaps contains the specified Swap
object.
Finally call the method in GameViewController.swift, inside handleSwipe(_:)
. Replace the existing handleSwipe(_:)
with the following:
func handleSwipe(_ swap: Swap) {
view.isUserInteractionEnabled = false
if level.isPossibleSwap(swap) {
level.performSwap(swap)
scene.animate(swap) {
self.view.isUserInteractionEnabled = true
}
} else {
view.isUserInteractionEnabled = true
}
}
Now the game will only perform the swap if it’s in the list of sanctioned swaps.
Build and run to try it out. You should only be able to make swaps if they result in a chain.
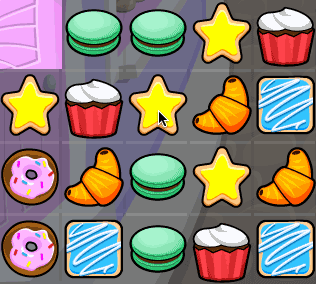
It’s also fun to animate attempted swaps that are invalid, so add the following method to GameScene.swift:
func animateInvalidSwap(_ swap: Swap, completion: @escaping () -> Void) {
let spriteA = swap.cookieA.sprite!
let spriteB = swap.cookieB.sprite!
spriteA.zPosition = 100
spriteB.zPosition = 90
let duration: TimeInterval = 0.2
let moveA = SKAction.move(to: spriteB.position, duration: duration)
moveA.timingMode = .easeOut
let moveB = SKAction.move(to: spriteA.position, duration: duration)
moveB.timingMode = .easeOut
spriteA.run(SKAction.sequence([moveA, moveB]), completion: completion)
spriteB.run(SKAction.sequence([moveB, moveA]))
run(invalidSwapSound)
}
This method is similar to animate(_:completion:)
, but here it slides the cookies to their new positions and then immediately flips them back.
In GameViewController.swift, change the else
clause inside handleSwipe(_:)
to:
scene.animateInvalidSwap(swap) {
self.view.isUserInteractionEnabled = true
}
Now run the app and try to make a swap that won’t result in a chain:
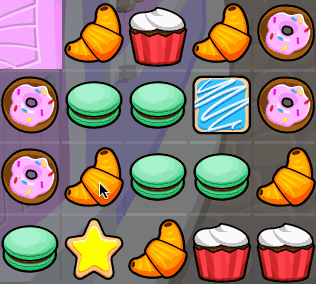
Where to Go From Here?
You can download the project to this point using the Download Materials button at the top or bottom of this tutorial.
Good job on finishing the second part of this tutorial series. You've done a really great job laying down the foundation for your game.
In part 3, you’ll work on finding and removing chains, refilling the level with new yummy cookies after successful swipes and keeping score.
While you take a well-deserved break, take a moment to let us hear from you in the forums.
Credits: Free game art from Game Art Guppy. The music is by Kevin MacLeod. The sound effects are based on samples from freesound.org.
Portions of the source code were inspired by Gabriel Nica's Swift port of the game.