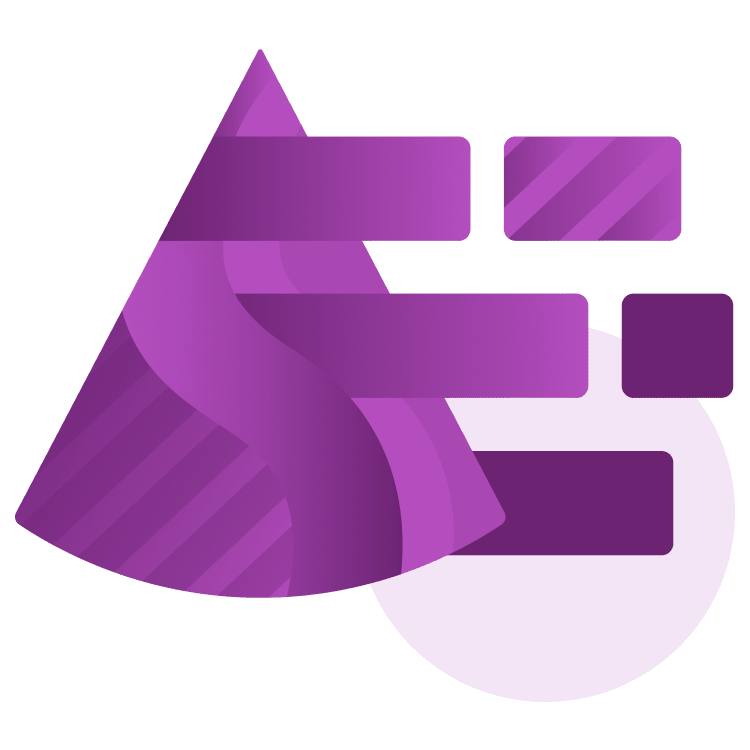
Introduction to Shaders in Godot 4
Discover the art of game customization with shaders in Godot 4. Learn to craft your visual effects, from texture color manipulation to sprite animations, in this guide to writing fragment and vertex shaders. By Eric Van de Kerckhove.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Introduction to Shaders in Godot 4
45 mins
- Getting Started
- What Is a Shader?
- Types of Shaders
- Basics of Texture Manipulation
- Fragment Shaders
- Overwriting Colors
- Manipulating Colors
- Vertex Function
- Moving Vertices
- Using Time
- Time to S(h)ine
- Selective Movement With If-Statements
- Circular Movement
- Getting the Dimensions of a Sprite
- Making Shaders Customizable
- Improving the Sway Shader
- Using Code to Set Shader Parameters
- Shader Hinting
- Combining Vertex and Fragment Functions
- Bonus Shaders
- Where to Go From Here?
Shader Hinting
In Godot, shader hinting is a feature to inform the engine how a shader parameter should be used. It allows you to specify that a parameter is a color or that its acceptable range lies between 25 and 50 for example.
To truly understand why you should keep shader hints in mind, you’ll be editing the solid_color shader to make it customizable.
Open the solid_color.gdshader file from the fragment folder in the shader editor. You created this shader at the start of the tutorial to color a sprite white. What if you wanted a different color though? You already know how to add that option by adding a uniform
variable to the shader. Like with the sway shader, you can move variables outside the functions and make them uniform
.
To make the color customizable, move the line below to the top of the shader, outside of the fragment
function:
vec4 new_color = vec4(1.0, 1.0, 1.0, 1.0);
Now make sure to add the uniform
keyword before it. The end result should look like this:
shader_type canvas_item;
uniform vec4 new_color = vec4(1.0, 1.0, 1.0, 1.0);
void fragment() {
...
}
Now try editing the color of the White node by selecting it, expanding its Material property, and changing the New Color parameter.
That doesn’t look like anything like a color selector! What gives?
It makes sense why you get X, Y, Z and W values here, because the color is stored in a vec4
variable. The X
value is the red component, the Y
value is the green component, the Z
value is the blue component and the W
value is the alpha component. While you can change the color this way, it’s not intuitive.
This is where you need a shader hint to tell the engine that this parameter is a color. To do that, add : source_color
after the name of the variable:
uniform vec4 new_color : source_color = vec4(1.0, 1.0, 1.0, 1.0);
This small change will tell Godot that the parameter new_color
is a color. Now take another look at the New Color parameter. It will now look a lot more inviting, with a nice color selector.
Feel free to make the sprite any color you like to test the shader.
You can find the full list of shader hints on the shading language page in the documentation.
Combining Vertex and Fragment Functions
Up until now you’ve been either using vertex
functions or fragment
functions. You can go a step further and combine them into a single shader! This way, you can create even more interesting effects.
To see how this works, you’ll be combining the sway shader with the grayscale shader. Add a new folder named combined to the shaders folder.
Now drag a tree.png sprite from the textures folder into the viewport and name the new node Combined. Now create a new shader called swaying_grayscale.gdshader in the combined folder. Next, open it in the shader editor.
Combining the two shaders is a matter of copying the code from both shaders. To start off, remove the existing vertex
function. Now copy the variables and the vertex
function from the sway shader and paste it into your new shader:
uniform float sway_amount = 20.0;
uniform float time_multiplier = 4.0;
void vertex() {
float sine_wave = sin(TIME * time_multiplier);
if (UV.y < 0.5) { // 4
VERTEX += vec2(sine_wave * sway_amount, 0);
}
}
The tree should now be swaying as expected.
Now copy the fragment
function from the grayscale shader and replace the existing fragment
function:
void fragment() {
vec4 input_color = texture(TEXTURE, UV);
float gray = 0.21 * input_color.r + 0.71 * input_color.g + 0.07 * input_color.b;
vec4 output_color = vec4(gray, gray, gray, input_color.a);
COLOR = output_color;
}
That’s it! You now have a grayscale tree that is swaying.
Bonus Shaders
I’ve added some extra shaders to the final project for you to try out and learn from. These don’t introduce any new concepts, but combine what you’ve learned here.
From left to right:
- Fade: Fades the alpha value of a sprite between 0.0 and 1.0 using the absolute value of a sine wave.
- Rainbow: Starts from a solid color and keeps adding to the red, green and blue components of the color to create a rainbow effect.
- Scrolling: Moves the UV coordinates of a sprite over time to create a scrolling effect.
You can find the source code for these shaders in the shaders / bonus_shaders folder. Each shader comes with comments explaining what each part does.
Where to Go From Here?
You can download the final project at top or bottom of this tutorial by clicking the Download materials link.
Congratulations on completing the tutorial, I hope I was able to inspire you to create your own shaders in Godot.
To learn more about shaders, check out the resources below:
- Godot shading language reference: Official documentation on the Godot shading language
- CanvasItem shaders: Official documentation on canvas item shaders
- The Book of Shaders: One of the best resources on shaders out there. This is a step-by-step guide to creating fragment shaders using GLSL.
- Godot version of the Book of Shaders: Someone converted all the GLSL shaders from the guide above to Godot shaders and made an application where you can live-edit them. I recommend taking a look at this alongside the guide above.
- Godot Shaders: A collection of Godot shaders created by the community.
Have fun experimenting with shaders in Godot!
If you have any questions or feedback, please join the discussion below.