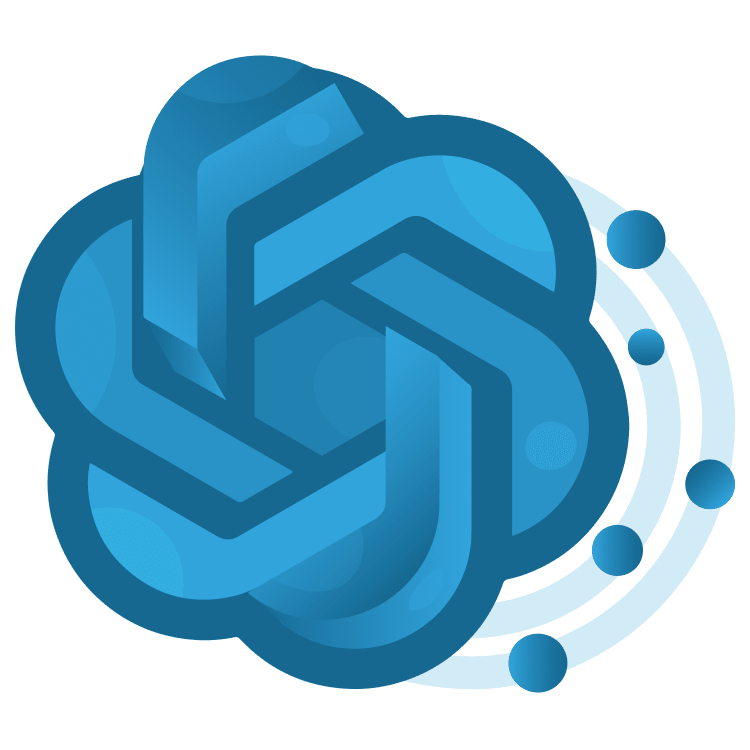
ChatGPT Tutorial for Flutter: Getting Started
Learn how to incorporate ChatGPT into your Flutter apps! In this tutorial, see how to leverage machine learning and ChatGPT with a real-world trivia app. By Alejandro Ulate Fallas.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
ChatGPT Tutorial for Flutter: Getting Started
30 mins
The tech space is all about AI and what it can do right now. Part of it is due to OpenAI’s ChatGPT, a chat app powered by their Generative Pre-Trained model. This enables you to draft documents, answer questions about a topic, or even write code.
In this tutorial, you’ll get started with ChatGPT by using it to build a simple trivia app called Wizz. Use OpenAI API as your app’s business logic provider instead of using custom backend services. In the process, you’ll learn the following:
- The basics of what ChatGPT is.
- Understand the capabilities of the GPT language model.
- To leverage the language model using best practices.
- To integrate ChatGPT-like features into your Flutter apps.
- How to deal with unexpected responses from the model.
Time to dive into the exciting world of AI!
Getting Started
Download the starter project by clicking the Download Materials button at the top or bottom of this tutorial. Then, open the starter project in VS Code. You can also use Android Studio, but you’ll have to adapt the instructions below as needed.
You should use a recent version of Flutter 3.10.1 or above. Make sure to get the latest dependencies for the project by running flutter pub get from your Terminal or directly from the VS Code prompt.
Looking at the code, you’ll find the starter project already provides some UI for the trivia app. Here’s a quick overview of the code structure:
- prompts.dart: This file will contain three prompts that’ll be sent to the model.
- data.dart: A layer that’ll interact with OpenAI’s API.
- domain.dart: Defines the different business logic models of Wizz, including Questions and Hints.
- pages: All screens are under this folder.
- widgets: Contains several parts of UI.
Build and run the project. You should see a screen asking for the player’s name, as shown below.
cd ios && pod install && cd ..
Type your name in the input and press Let’s Wizz Up to start a new game.
The trivia game is incomplete since the game screen is just a placeholder. You’ll add the core features for Wizz throughout the tutorial.
Understanding ChatGPT’s Capabilities
One of the most famous AI-powered apps is ChatGPT. In simple terms, ChatGPT is an AI system created and trained by OpenAI to understand and generate human language.
The last three letters in the name stand for Generative Pre-Trained Transformers. GPT refers to a broader family of language models based on a specific architecture. These models are trained on a large amount of data to generate human-like content, such as text, images or even music.
GPT-based models can usually help you build applications that allow you to:
- Write documents.
- Answer questions.
- Analyze text.
- Create an interactive chatbot.
- Translate different languages.
- Generate code.
- Solve bugs in a code block.
- And so much more.
ChatGPT is a specific implementation of a GPT model. OpenAI built it for having chat-based human conversations. When integrating it into your app, understanding the difference between ChatGPT and its underlying GPT model is essential.
The main difference between ChatGPT and GPT lies in their intended use cases and design considerations. OpenAI designed ChatGPT to engage in back-and-forth conversations with users. GPT models rely on prompts to generate responses. These prompts set the tone and provide context for the generated text. In contrast, ChatGPT was optimized for dialogues for a back-and-forth conversation.
In general, you can think of ChatGPT as an app and GPT as the brains behind the app.
OpenAI launched an API to enable developers to add GPT-based features to apps or even create new apps based on the model’s features. This, in turn, helps them retrain their model and improve the quality of the generated text outputs.
Now that you’ve learned these basic concepts about AI and language models, it’s time to add GPT-based features to Wizz, a trivia game that only wizards (pun intended) can beat!
Obtaining an OpenAI API Key
To add AI to your app, you’ll use OpenAI’s API. For this, you’ll need an account. You can create a new one or use your own.
To create a new account, open your web browser and navigate to https://platform.openai.com/overview. Use the Sign Up button on the top right corner or use Log In if you already have an account.
You’ll need the following information to create an account:
- Password
- Firstname
- Lastname
- Birthdate
- Working phone number – you’ll need it for verifying your information
After you’ve created your account, go ahead and log in. You should see something like this:
Now, click on your profile, again located in the top right corner of the screen. It should display a pop-up menu like the following:
Then, select View API keys. Next, press Create new secret key. You can choose a name for the key (it’s optional) and click Create secret key. This will create your secret key.
Make sure to copy the key somewhere safe since you won’t be able to view it again. You’ll need to add this key to the project later on.
Great job, you’ve created an account and have access to OpenAI’s API platform. This is a small step for your app but a giant leap for your development skills.
Learning Best Practices for GPT Models
Now that you’ve taken the first step towards implementing AI in your apps, it’s time to discuss best practices when interacting with GPT models via API or the ChatGPT app.
Although GPT models do a great job of trying to understand what you mean, they might not always work as expected since it can’t read your mind (yet!).
This is where best practices around prompting come in. They’ll help you get better results from the language model.
Here are some strategies that might improve your results when using GPT models. Each of them also has examples that you can play around with in OpenAI’s Playground:
You’ll provide questions for the game and give hints to the players.
When providing questions, you’ll add humor to the question in the form of a fun fact.
When giving fun facts, you should add puns to make it fun.
These scenarios are a great example of when to make it easier on the model by breaking them down step by step. For example, when generating trivia questions, you might want the model to follow certain steps. Here’s the guide you’ll use when prompting the model for new trivia questions:
To solve this, do the following:
– First, you’ll randomly select one of the following topics: history, arts, entertainment, sports, science, or geography.
– Then, you’ll generate a question based on the topic selected.
To solve this, do the following:
– First, you’ll analyze the question and determine the correct answer.
– Then, you’ll generate a hint that’ll make it easier to answer. Make sure that the hint is not too obvious.
Don’t decide on the hint until you’ve answered the question yourself.
-
Use persona to give context: Sometimes, the answer you get might not be what you wanted in terms of domain or technical depth. In those cases, it might be a good idea to give a persona definition to help you get the answer you are looking for. For example, here’s the persona that you’ll use to ensure that the model knows what style of responses you are expecting:
You are the know-it-all and funny host of a trivia game called Wizz.
-
Give step-by-step guides to complete a task: Some tasks can be complex enough that the model can have difficulty understanding them.
Your task is to generate a new trivia question for the player.
-
Provide output examples: If you want the model to copy a particular style or follow a certain format when responding. Then it’s a good idea to provide examples. You can give an example of the voice and tone of the response or even a JSON template to follow. For example, when interacting with the model via API, you’ll usually want to end your prompts with an example of the JSON expected in the response, like below:
Your output should only be JSON without formatting, and follow this template:
{ “action”: “player_hint”, “hint”: “” } -
Give GPTs time to figure out their own solution: Sometimes, you’ll get better results when instructing the model to reason first before giving an answer. This is a common scenario when validating answers from a problem statement given by other users. A good use case in Wizz is when asking for hints, you’ll first want the model to understand the question before giving a hint so that you avoid obvious hints.
Your task is to give a hint to the player without giving away the answer.
{ “action”: “player_hint”, “hint”: “
These are a few tactics you can use to improve your results, but more tactics are available for different scenarios. Head over to OpenAI’s best practices documentation for more information. You can use OpenAI’s Playground to test your prompts and play around with the model. This is a great way to test your prompts before integrating them into your app’s code.