Moving Targets
In this game, we don’t actually want our ninja to move, so comment out this line:
self.player.moveVelocity = GLKVector2Make(50, 50);
Instead, we want to add some moving targets into our scene for our ninja to combat. We’ll create them offscreen to the right, and move them to the left.
To do this, add this new method to SGGViewController.m, right before the update method:
// Add new method before update method
- (void)addTarget {
SGGSprite * target = [[SGGSprite alloc] initWithFile:@"Target.png" effect:self.effect];
[self.children addObject:target];
int minY = target.contentSize.height/2;
int maxY = 320 - target.contentSize.height/2;
int rangeY = maxY - minY;
int actualY = (arc4random() % rangeY) + minY;
target.position = GLKVector2Make(480 + (target.contentSize.width/2), actualY);
int minVelocity = 480.0/4.0;
int maxVelocity = 480.0/2.0;
int rangeVelocity = maxVelocity - minVelocity;
int actualVelocity = (arc4random() % rangeVelocity) + minVelocity;
target.moveVelocity = GLKVector2Make(-actualVelocity, 0);
}
Here we create a new target and add it to the list of children so it will be rendered and updated each frame.
We then figure out where to position the target when it spawns. We place it offscreen to the right, at a random spot between the bottom and top of the screen.
Then we figure out how fast it should move. We pick a random value between 480.0/4.0 (the width of the screen in 4 seconds) and 480.0/2.0 (the width of the screen in 2 seconds), going to the left.
Next make the following changes to SGGViewContorller.m:
// Add new property in private @interface
@property (assign) float timeSinceLastSpawn;
// Synthesize property
@synthesize timeSinceLastSpawn = _timeSinceLastSpawn;
// Add to beginning of update method
self.timeSinceLastSpawn += self.timeSinceLastUpdate;
if (self.timeSinceLastSpawn > 1.0) {
self.timeSinceLastSpawn = 0;
[self addTarget];
}
Here we just add some code to call addTarget every second.
Compile and run, and now you have some targets running across the screen!
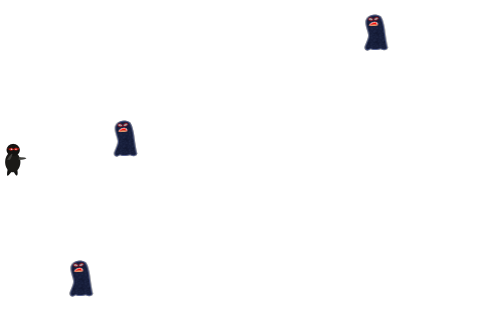
Where To Go From Here?
Here is an example project with all of the code from the tutorial so far.
Congratulations, you have made the start of a game using the lowest-level APIs on iOS – OpenGL ES 2.0 and GLKit! You have drawn sprites to the screen, positioned them where you like, and even made them move.
You’re ready for the next tutorial in the series, where you’ll let the ninja shoot stars, kill monsters, and win the game.
In the meantime, if you have any questions or comments, please join the forum discussion below!

This is a blog post by site administrator Ray Wenderlich, an independent software developer and gamer.