Displaying the Score
Your game’s looking pretty good now! All that’s left is to display the score.
For this game, you’re going to implement a dynamic way of showing the score. Instead of having a fixed display at the top or bottom of the screen, you’re going to show the score in a temporary pop-up window each time the player scores.
Begin by adding the following instance variable to HelloWorldLayer.h:
CCLabelTTF *scoreText;
Now add this new method to HelloWorldLayer.mm:
-(void)setupScore {
score = 0;
CGSize winSize = [[CCDirector sharedDirector] winSize];
scoreText = [CCLabelTTF labelWithString:@"Score: 0"
fontName:@"Arial"
fontSize:22
dimensions:CGSizeMake(200, 50)
hAlignment:kCCTextAlignmentLeft
vAlignment:kCCVerticalTextAlignmentCenter];
scoreText.color = ccWHITE;
scoreText.position = ccp(100, winSize.height-25);
[self addChild:scoreText z:20];
}
The above method creates a new label and assigns it to the scoreText variable. It then adds this label to the game layer and sets the label position to be the top left corner.
Now call this new method from init right after the call to setupAudio:
[self setupScore];
This is good, but right now you’re not showing the actual score to the user. You’re just showing some text. Let’s make the text change when the user scores.
Replace the current implementation for scoreHitAtPosition:withPoints: with this:
-(void)scoreHitAtPosition:(CGPoint)position withPoints:(int)points {
score += points;
[scoreText setString:[NSString stringWithFormat:@"Score: %d", score]];
NSString* curScoreTxt = [NSString stringWithFormat:@"+ %d", points];
CCLabelTTF *curScore = [CCLabelTTF labelWithString:curScoreTxt fontName:@"Marker Felt" fontSize:24];
curScore.color = ccWHITE;
curScore.position = position;
[self addChild:curScore z:20];
id opacityAct1 = [CCActionTween actionWithDuration:1 key:@"opacity" from:255 to:0];
id actionCallFunc = [CCCallFuncN actionWithTarget:self selector:@selector(removeScoreText:)];
id seq = [CCSequence actionOne:opacityAct1 two:actionCallFunc];
[curScore runAction:seq];
}
This adds the new points to the score variable. Then it sets the text of your score label to be the newly updated score.
You then create a new label that will be displayed at the position you received via the position parameter. As shown in the code, this is the position of the coin or bunny triggering the score update. The coin or bunny vanishes upon collision with the player, and a score display temporarily appears in its place.
You add the new label to the layer and then create two actions. The first action makes the new score label disappear after one second by changing the opacity property of the sprite from 255 (full visibility) to 0 (not visible). The second action calls a new method, removeScoreText:.
Finally, you create a sequence using the two defined actions and run that sequence on the new label sprite.
You still need to add the removeScoreText: method called by the second action. Add it as follows:
-(void)removeScoreText:(CCLabelTTF*)scoreLabel {
[scoreLabel removeFromParentAndCleanup:YES];
}
This new method just removes the score display from the layer.
Run the game now and see how nicely this works. Every time the player makes contact with a coin or a bunny, a label pops up showing the updated score, displays for one second, then disappears.
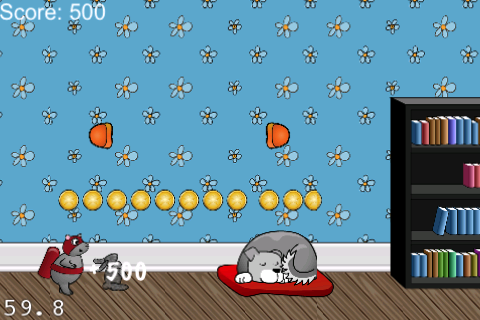
There’s nothing left to do but to run the game and enjoy!
Where to Go From Here?
Congratulations, you’ve finished the game! It’s been a long journey. I hope you enjoyed creating this wonderful game with me, and hardly noticed you were learning in the process. Thanks for reading!
A project with all of the code as at the end of the tutorial series can be found here.
As always, I am available to answer questions about this tutorial series in the forums, both here and on the LevelHelper forum.
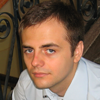
This is a post by special contributor Bogdan Vladu, an iOS application developer and aspiring game developer living in Bucharest, Romania.