Testing a Failed Response
For this test, you’ll create a response that returns after five seconds. You’ve configured the app to request timeout after three seconds so it will treat a five-second response as a failure.
Add the following method to MainActivityTest.kt and import dependencies:
@Test
fun testFailedResponse() {
mockWebServer.dispatcher = object : Dispatcher() {
override fun dispatch(request: RecordedRequest): MockResponse {
return MockResponse()
.setResponseCode(200)
.setBody(FileReader.readStringFromFile("success_response.json"))
.throttleBody(1024, 5, TimeUnit.SECONDS)
}
}
val scenario = launchActivity<MainActivity>()
onView(withId(R.id.progress_bar))
.check(matches(withEffectiveVisibility(ViewMatchers.Visibility.GONE)))
onView(withId(R.id.meme_recyclerview))
.check(matches(withEffectiveVisibility(ViewMatchers.Visibility.GONE)))
onView(withId(R.id.textview))
.check(matches(withEffectiveVisibility(ViewMatchers.Visibility.VISIBLE)))
onView(withId(R.id.textview))
.check(matches(withText(R.string.something_went_wrong)))
scenario.close()
}
In the code above, throttleBody()
throttles the response by five seconds. This test verifies one extra thing: It checks whether the view with ID R.id.textview
contains the text that the string resource R.string.something_went_wrong
refers to.
Run MainActivityTest
. You’ll see that both tests pass.
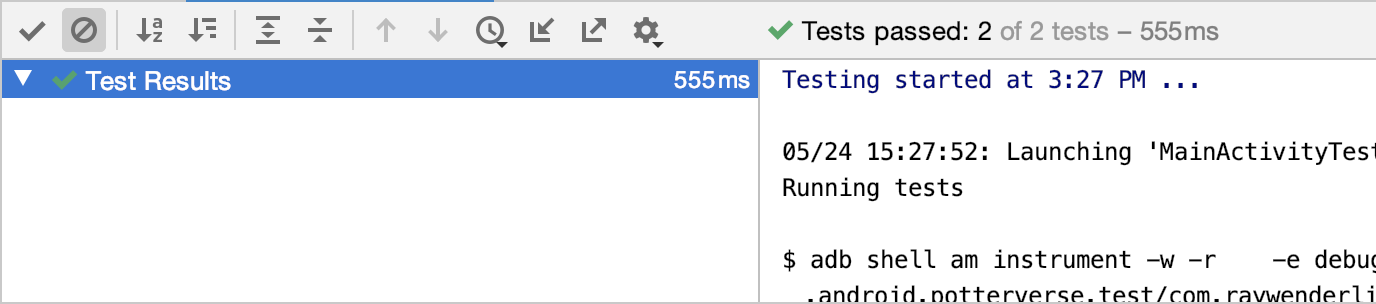
Congratulations! You’ve now set up tests to check your app’s success and failure responses on a mock web server.
Where to Go From Here?
Download the final project by using the Download Materials button at the top or bottom of this tutorial.
In this tutorial, you’ve set up MockWebServer, configured it to mock API calls from a remote server and written UI tests to verify how an app functions.
As a further enhancement, make the app display a text saying Oops! Seems like a server error when the API returns a status code of 500. Configure MockWebServer to return a 500 status code and write a UI test to verify that the app displays the text.
To learn more about MockWebServer, refer to the documentation on the MockWebServer repository.
Hopefully, you’ve enjoyed this tutorial! If you have any questions or ideas to share, please join the forum discussion below.