Finishing Touches
Your game is fully functional, and very near completion. However, why not add two methods to make a truly ~classy~ app.
The first method will be used to print the user's health. You can then call this method after every day passes to provide the user with a health update. The second method will be purely for educational purposes (not super-classy).
Declare a method called printHealth in Game.h:
-(void)printHealth;
As you'll notice, the method has no return value or any parameters, so it will look like every other method you've created up until this point. The function just needs to log the player's name and print their health. Do that via the following code added to the end of Game.m (above the @end):
-(void) printHealth
{
if (health>0) {
NSLog(@"\n\n%@ managed to finish the day with a total health of: %i\nPress enter to continue.", name, health);
waitOnCR();
}
}
Note that I included an if condition in the above code because I thought it would be strange to log a user health of 0, especially when they should have died. Call printHealth either at the end of day1, day2, and day3 or after each call to day1, day2, and day3 in section #4 of main in main.m.
Build and run to test!
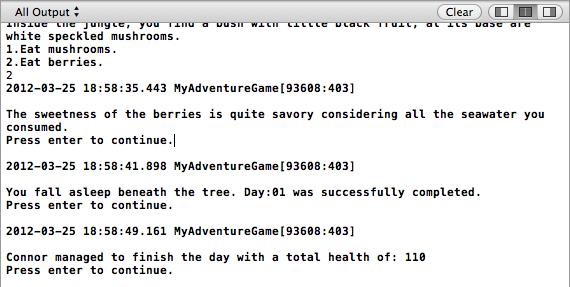
Your final method is unique because it takes a parameter. You want to create a method that logs the player's score based on what percentage of health points they get out of the overall possible health points. The parameter will be how many health points the user received.
Create another integer instance variable named "score" in Game.h:
int score;
Add a property declaration for it:
@property int score;
And synthesize it in Game.m:
@synthesize score;
The method will be called printScore(x). Add the declaration to Game.h:
- (void) printScore: (float) x;
You'll note that while the method returns void, it takes an argument x, which is a variable of type float.
And here's the implementation of printScore(x) in Game.m (add it to the end above @end):
-(void) printScore:(float) x
{
score = (x/110) * 100;
NSLog(@"%@ based on your health you received %i percent of the available points.", name, score);
waitOnCR();
}
In order to use the above method, you have to call it and provide the number of health points (x) received and since the health points is available via the health property, we can simply pass that. Try calling the printScore: method right before section #5 in main in main.m as follows:
[myGame printScore:myGame.health];
Where to Go From Here?
This concludes the second tutorial in the beginning programming for iOS series for high school students. Click here to download the full project.
I hope you come away from this tutorial with a basic understanding of arrays, classes, objects, methods and properties. If you feel confident, try messing around with the text-based survival game format provided here to create your own scenarios. For example:
- If the game went on long enough, can you make it so that the user could lose enough health cumulatively to die? You could try subtracting a small amount of health every morning of each new day, for example, to account for the effects of lack of food and exposure to the elements.
- Try implementing dialogue into the game, where the user is given choices of how to respond to, say, a talking monkey or a native who knows English.
- Create a variable that can store food or water the user finds on the island, so that the user has a way to increase health if it gets dangerously low. Give the user the choice of consuming the food or water after each decrease in health.
In the meantime, I'm looking forward to reading your feedback in the forums. And stay tuned: the next tutorial will be an iPhone app!