Removing Unconnected Caverns
If you choose to remove all the disconnected caverns, then you need to fill them in with wall tiles.
To do that, add this new method to Cave.m:
- (void) removeDisconnectedCaverns
{
NSInteger mainCavernIndex = [self mainCavernIndex];
NSUInteger cavernsCount = [self.caverns count];
if (cavernsCount > 0) {
for (NSUInteger i = 0; i < cavernsCount; i++) {
if (i != mainCavernIndex) {
NSArray *array = (NSArray *)self.caverns[i];
for (CaveCell *cell in array) {
((CaveCell *)self.grid[(NSUInteger)cell.coordinate.y][(NSUInteger)cell.coordinate.x]).type =
CaveCellTypeWall;
}
}
}
}
}
First, this method gets the index of the main cavern, then it uses a for
loop to go through the caverns
array. It turns all the CaveCell
instances into walls within the caverns, except for those in the main cavern.
To see your latest efforts in action, add the following line of code to generateWithSeed:
just before the line that calls generateTiles
:
[self removeDisconnectedCaverns];
Build and run now, and note that your cave no longer has any unconnected caverns.
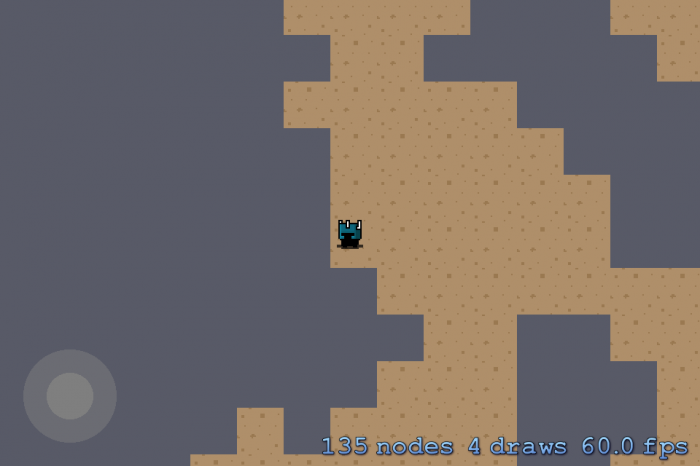
Tip: It might be difficult to actually see what has changed between runs. The following illustrations show exactly what happened.
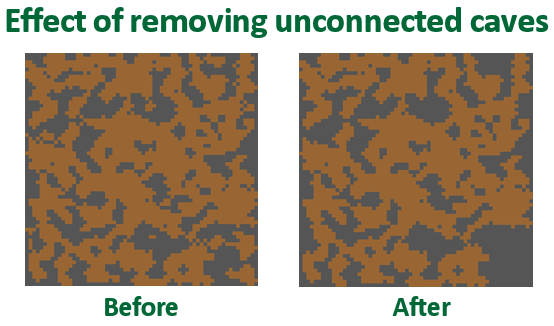
These images were created by using
spriteNodeWithColor:size:
with a size of 2x2 points in place of using
spriteNodeWithTexture:
in
generateTiles
, and modifying
sizeOfTiles
accordingly.
Where To Go From Here?
Here is the finished example project from this tutorial series so far.
Congratulations, you have generated your own random cave system using cellular automata! Your knight now has a mysterious cavern to explore.
However, there's more you can do! Stay tuned for the second and final part of the series, where you'll learn how to connect unconnected caverns, place an exit and entrance into the cavern, add collision detection - and yes, there will be treasure.
In the meantime, if you have any questions or comments, please join the forum discussion below!